Okay, here’s my take on sharing my “double jump ring” practice and journey, blog-style:
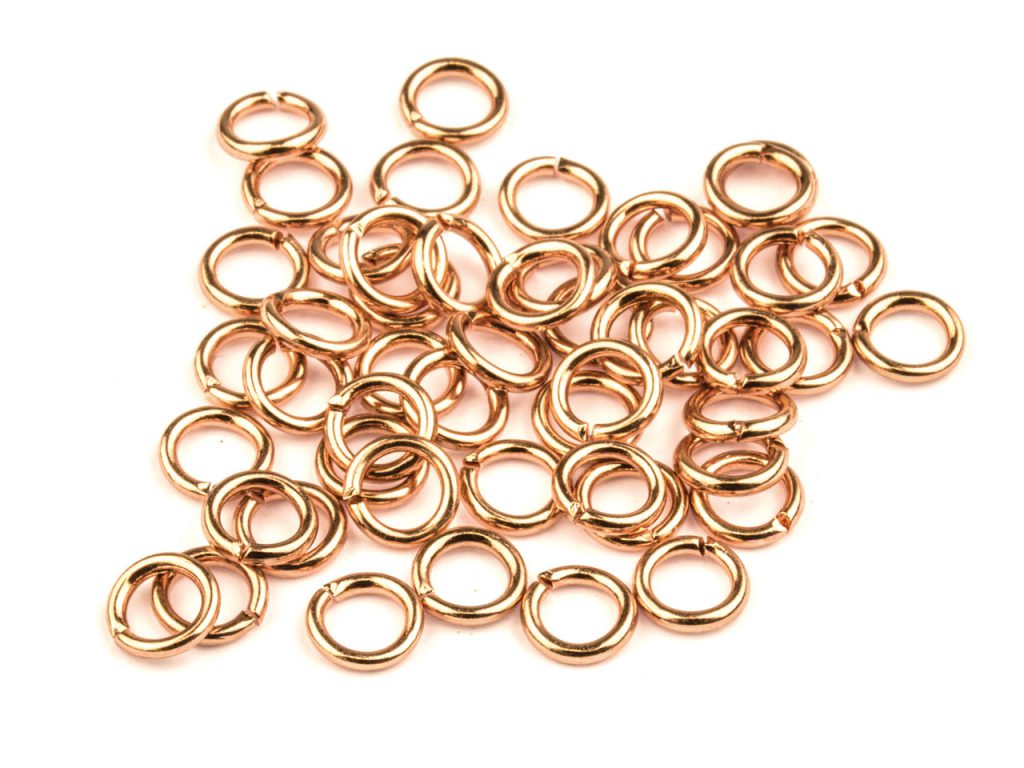
Hey everyone! So, been messing around with some game mechanics lately, and I finally got something kinda cool working: a double jump ring! Thought I’d share how I did it, ’cause why not?
First things first, the idea. I wanted a ring item that, when equipped, gives the player a double jump. Sounds simple, right? Well, kinda.
Started with the player. I had to make sure the player character could double jump in the first place. So, I dug into the player movement script. I added a variable to track if the player has already jumped once while in the air, something like hasDoubleJumped
. Set it to false
when the player is grounded.
Then, in the jump logic, I checked:
- Is the jump button pressed?
- Is the player grounded, OR have they not double jumped yet (
!hasDoubleJumped
)?
If both are true, then jump! If they weren’t grounded then also set hasDoubleJumped = true;
after they jumped.
Finally, add the reset. When the player lands, the hasDoubleJumped
variable needs to be set back to false
.
Next up, the ring itself. I created a new scriptable object for items. This scriptable object holds all the basic item data: name, description, icon, and importantly, what it does. I added a boolean flag to the scriptable object, like grantsDoubleJump
. If this is true, then wearing the ring should give you a double jump, and not, it shouldn’t.
Equipping the ring. This part was a little more involved. I have an equipment system in my game. When the player equips an item, I need to check its scriptable object and if grantsDoubleJump
is true, then somewhere on the player there needs to be some sort of variable that says, “Hey I have a double jump now!”. I added something to the player’s stats script and called it canDoubleJump
. A nice simple boolean.
I then modified the player’s jump logic to only use the double jump code if canDoubleJump
is true.
It basically changes my previous check from !hasDoubleJumped
to canDoubleJump && !hasDoubleJumped
Putting it all together. I made an actual ring item and set its grantsDoubleJump
flag to true. I equipped the ring and then jumped. Success! The player character performed a beautiful double jump. Took it off, and the character could no longer double jump.
Challenges and Tweaks
Timing. The first version felt kinda clunky. The double jump felt delayed after the first jump. I messed with the jump force and the timing to make it feel snappier. I also added a small visual effect (a little puff of smoke) when the player double jumps, just to give some feedback.
Multiple Rings? What if the player could equip two rings that both granted double jumps? I didn’t want them to get a triple jump by accident! I just made the canDoubleJump
a simple boolean, so it doesn’t matter how many rings give you a double jump, it will still only ever be 1 double jump available.
Future Stuff. I’m thinking of adding different kinds of double jumps. Maybe a ring that grants a longer double jump, or one that lets you change direction mid-air. Plenty of room to play around!
Anyway, that’s how I made a double jump ring. It was a fun little project, and I learned a thing or two along the way. Hope this was helpful or interesting to someone! Cheers!