Okay, so today I’m gonna walk you through this little side project I did – a League of Legends ban check tool. Nothing super fancy, but it scratched an itch I had and I figured someone else might find it useful, or at least find the process interesting.
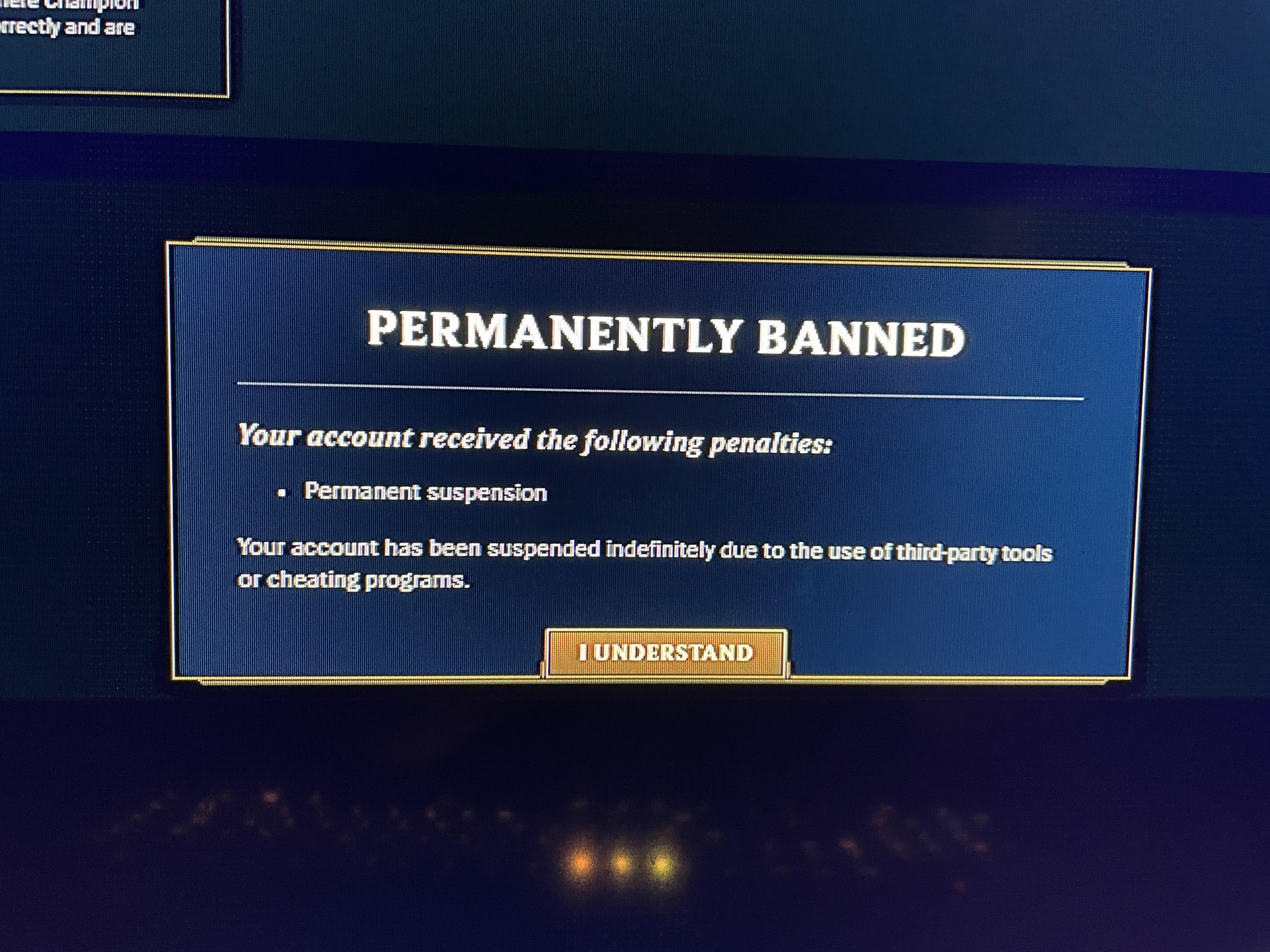
It all started ’cause I was tired of dodging games in champ select. You know how it is, you get into a lobby and someone’s already instalocked Yasuo mid with a 40% win rate? Or maybe someone is first timing a champ in ranked. I wanted a quick way to check everyone’s stats before the game started so I could make a more informed decision about dodging. Less LP loss, you know?
First, I had to figure out how to get the data. Riot’s API is the obvious choice, but it’s a bit of a pain to get started. I spent a good chunk of time just figuring out the authentication process and getting my API key. Pro tip: read the documentation carefully. I skimmed it the first time and wasted an hour because I missed a crucial step. Learn from my mistakes!
Next up: figuring out the API endpoints. Riot’s API is pretty well-documented once you get the hang of it, but there are a lot of endpoints. I needed to find the ones that would give me the summoner’s name, their ranked stats, and their recent match history. It took some trial and error, and a lot of * debugging, but eventually I found the right ones.
Once I had the data flowing, I needed to actually do something with it. I decided to use Python, mostly because I’m most comfortable with it. I used the `requests` library to make the API calls and `json` to parse the responses. I also used `pprint` just to make the output a little easier to read while I was developing.
Here’s a simplified version of the code I ended up with:
- First, you have to use your api key after you get it.
- Second, you have to use the summoner name of any player you want to inspect.
python
import requests
import json
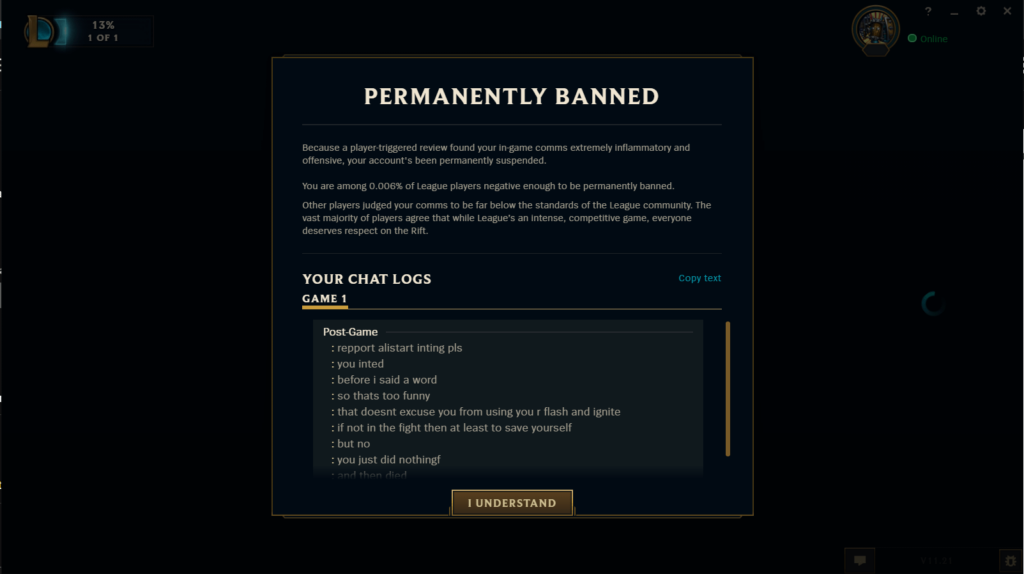
from pprint import pprint
API_KEY = “YOUR_API_KEY” # Replace with your actual API key
def get_summoner_info(summoner_name):
url = f”*.com/lol/summoner/v4/summoners/by-name/{summoner_name}?api_key={API_KEY}”
response = *(url)
*_for_status() # Raise HTTPError for bad responses (4XX or 5XX)
return *()
def get_ranked_stats(summoner_id):
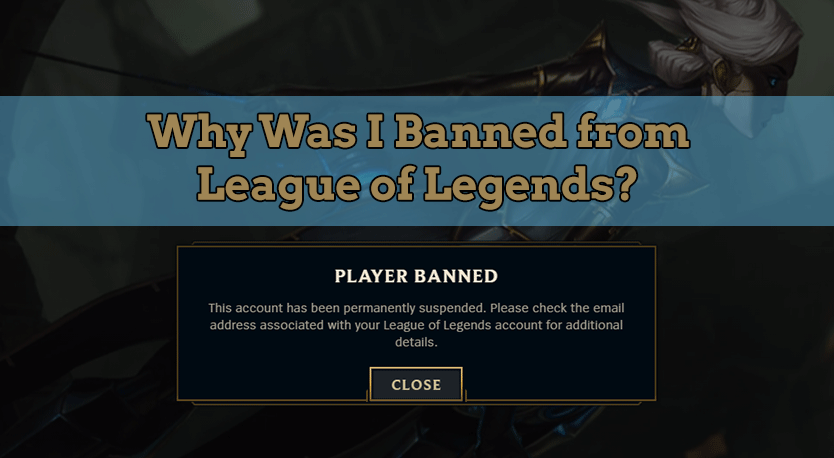
url = f”*.com/lol/league/v4/entries/by-summoner/{summoner_id}?api_key={API_KEY}”
response = *(url)
*_for_status()
return *()
def main():
summoner_name = input(“Enter summoner name: “)
try:
summoner_info = get_summoner_info(summoner_name)
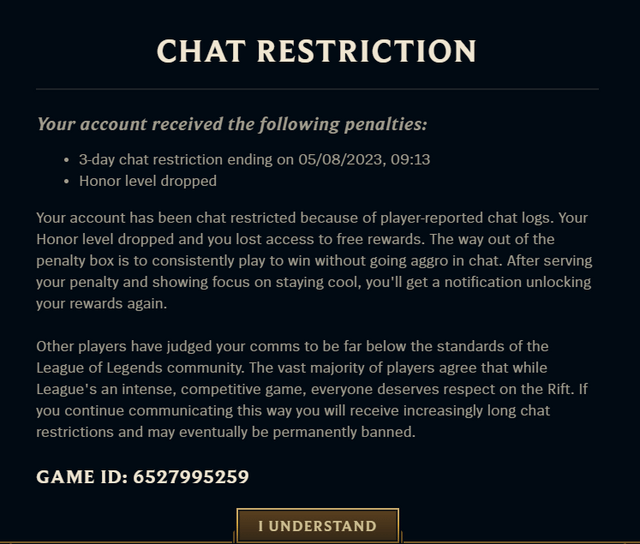
summoner_id = summoner_info[‘id’]
ranked_stats = get_ranked_stats(summoner_id)
print(f”Summoner Name: {summoner_info[‘name’]}”)
if ranked_stats:
for queue in ranked_stats:
print(f” Queue Type: {queue[‘queueType’]}”)
print(f” Rank: {queue[‘tier’]} {queue[‘rank’]}”)
print(f” LP: {queue[‘leaguePoints’]}”)
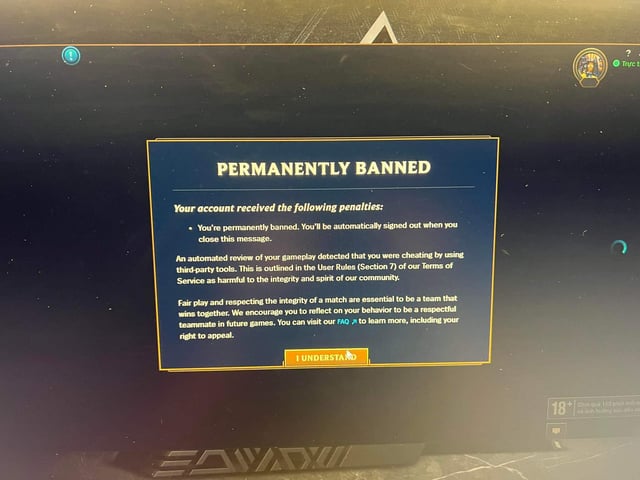
print(f” Wins: {queue[‘wins’]}”)
print(f” Losses: {queue[‘losses’]}”)
else:
print(” Unranked”)
except * as e:
print(f”Error: {e}”)
except KeyError as e:
print(f”Error: Could not find key: {e}”)
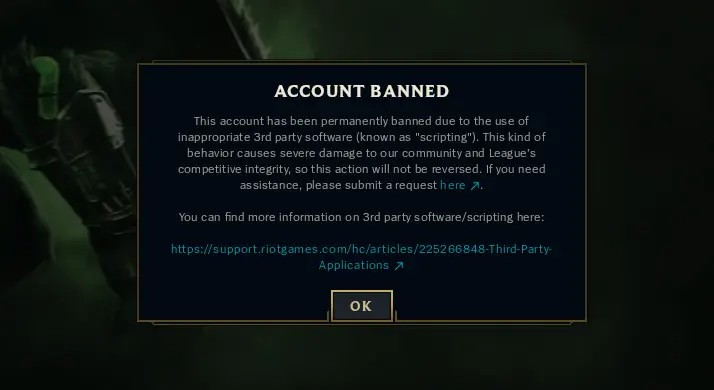
except Exception as e:
print(f”An unexpected error occurred: {e}”)
if __name__ == “__main__”:
main()
This script takes a summoner name as input, fetches their summoner ID, and then retrieves their ranked stats. It prints out the summoner’s name, their rank in each queue, their LP, and their win/loss ratio. Pretty basic, but it’s a start.
The error handling isn’t perfect. Riot’s API can be flaky sometimes, and I didn’t want the whole script to crash if it just couldn’t find a summoner or something. The `try…except` blocks catch common errors like HTTP errors and missing keys in the JSON response.
So, that’s it! It’s not the most polished or feature-rich tool, but it does the job. I’ve found it helpful for making those last-second dodge decisions. Maybe it’ll be helpful for you too, or at least give you some ideas for your own projects. Happy coding!