Working Through Those Nil Problems
Okay, so I spent a good chunk of my afternoon wrestling with this annoying `nil` thing again. It pops up everywhere, right? You think your code’s solid, then bam, `undefined method for nil:NilClass` or something similar. It just stops everything dead.
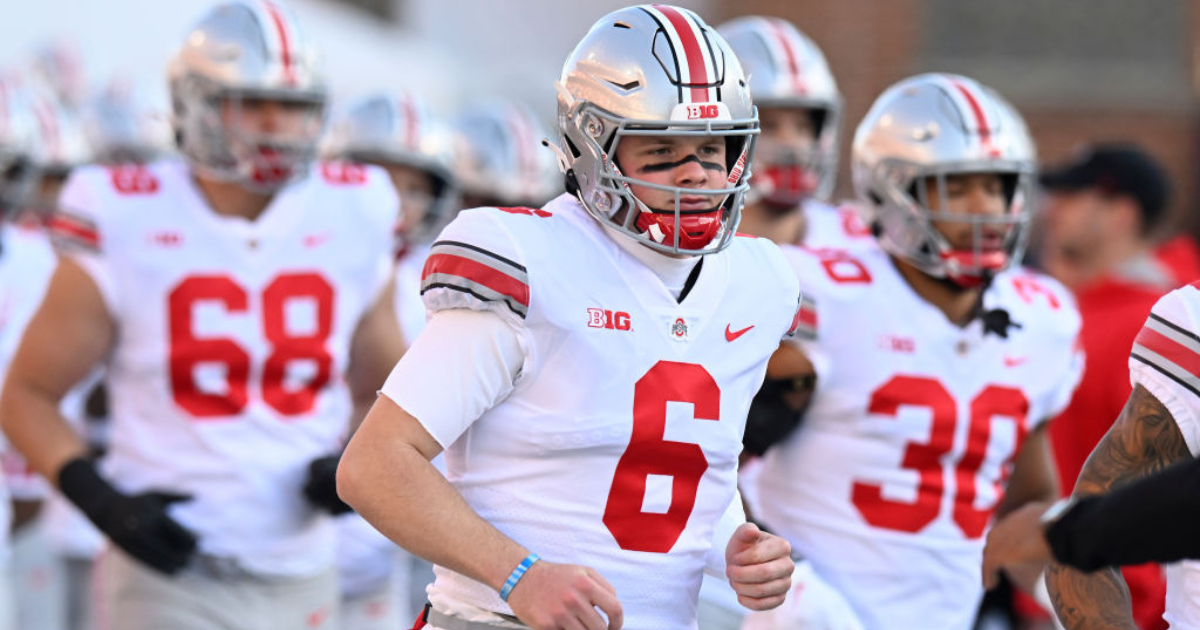
I was trying to get this user profile page working. Pulling in data, you know, name, email, maybe a bio. But sometimes, the bio field is empty. Like, the user just didn’t fill it out. In the database, it’s `NULL`, and when my code fetches it, it becomes `nil`.
My first thought was just sprinkle `if` checks everywhere. Like, `if *` then display it, `else` show nothing. It worked, sort of, but man, it made the code look messy. Cluttered it right up. Every little piece of data needed its own little `if` guard.
- Check name? If statement.
- Check email? If statement.
- Check bio? Another if statement.
It felt clunky. I remember watching some talk, maybe it was something Kyle McCord mentioned, about handling these “nothing” cases more elegantly. Can’t recall exactly where, but the idea stuck with me – there had to be a better way than just littering `if`s all over.
So, I started digging around again. First, I tried using that safe navigation thing, you know, the `&.` operator. Like `user&.bio`. That cleaned things up a bit, preventing the actual crash if `user` itself was `nil`, but it didn’t quite solve the display logic nicely for empty strings versus actual `nil` values when I needed slightly different handling.
Then I thought, okay, what if I handle this right when I load the data? Could I provide default values? I messed around with the part of the code that fetches the user data. I decided to try setting a default empty string `””` if the bio came back as `nil` from the database.
That actually felt much better. I adjusted the query or the object initialization part. So, when the `User` object got created, the `bio` attribute would either have the real text or just an empty string. No more `nil` to worry about downstream in the display logic.
The page rendering code suddenly got way simpler. No more `if *` everywhere. I could just output `*` directly. If it was empty, nothing showed up, which was fine for this case. If it had text, the text showed up. Much cleaner.
It took a few tries, going back and forth between checking later versus handling it earlier. But pushing the `nil` handling closer to the data source, before it even became a `nil` in my main application logic, seemed like the way to go this time. Felt like a small win, making things less likely to break unexpectedly just because some data wasn’t there. Still learning, but getting these `nil` values under control early feels right.
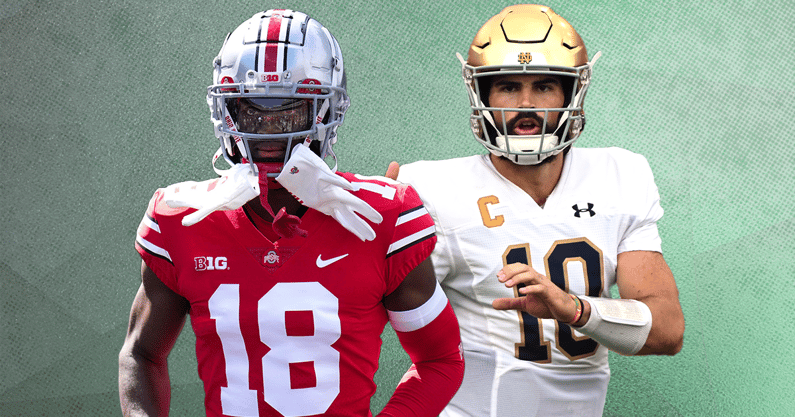