Okay, so I wanted to make a simple leaderboard for my game, just something to show the top scores. I’ve seen a few tutorials, but they were all overly complicated for what I needed. I just wanted something basic using Creator Classic, you know?
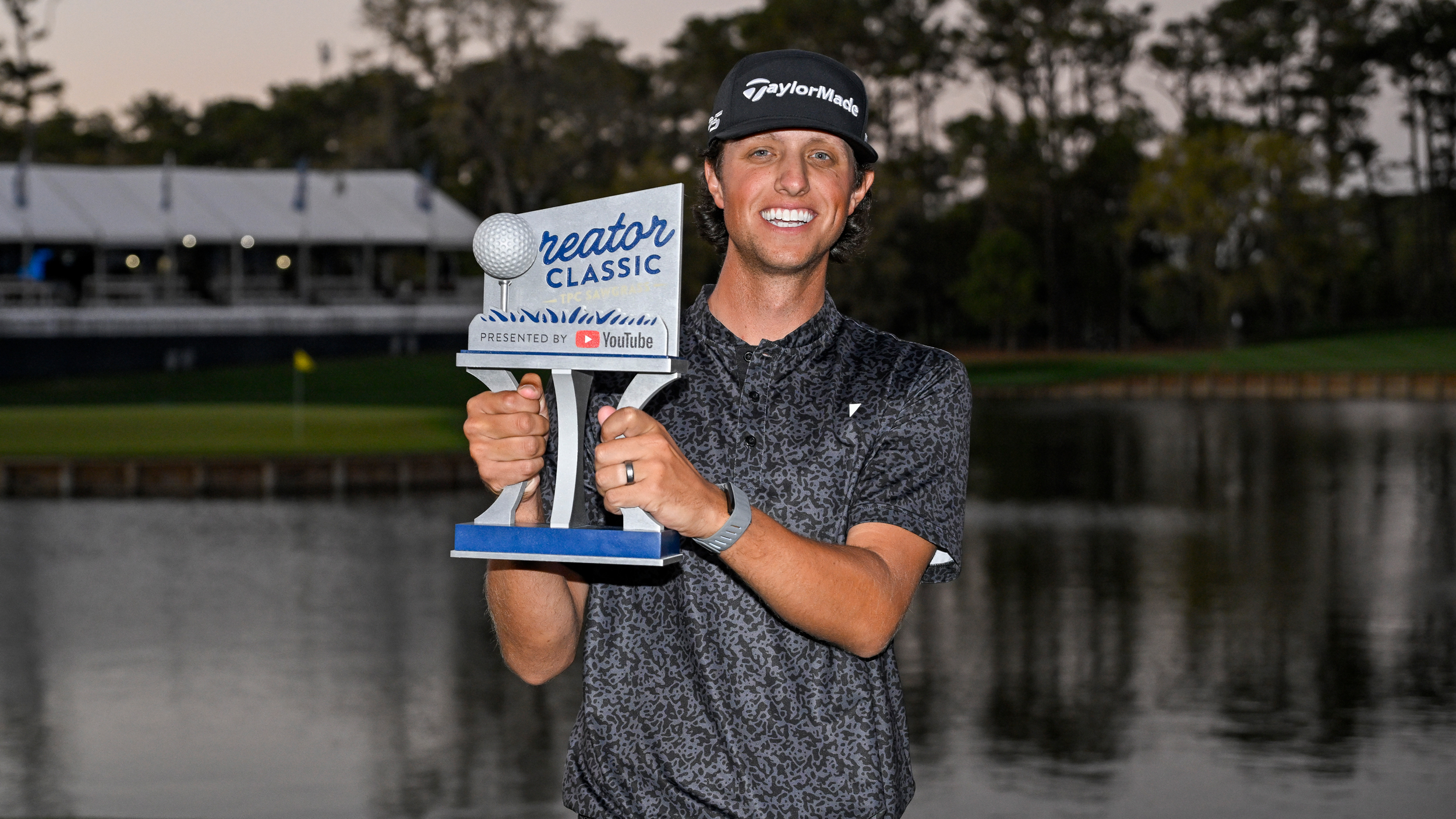
First, I created a new project in Creator. I just went with the basic 2D template, nothing fancy.
Setting up the UI
Next, I needed to set up the UI. This is where the leaderboard would actually display.
- I added a Canvas to my scene. This is pretty standard for any UI stuff.
- Then, I added a Scroll View. This way, if I had a ton of scores (wishful thinking!), they wouldn’t just run off the screen.
- Inside the Scroll View, I added a Content object. This is where the individual score entries would go.
- I made a Prefab for the score entry. This was just a simple Text object to display the player’s name and score. I made sure to set the text to be a decent size and readable.
The Scripting Part
Now for the code! I created a new script called “LeaderboardManager”. This would handle adding scores, sorting them, and displaying them.
I started by defining a simple class to hold the score data:
Then, in my LeaderboardManager, I created a list to store these ScoreEntry objects.
I wrote a function called “AddScore” that would take a player’s name and score, create a new ScoreEntry, add it to the list, and then call another function to update the display.
The “UpdateDisplay” function was the trickiest part. I needed to:
- Sort the list of scores in descending order (highest score first). I used a simple LINQ query for this.
- Clear out any existing entries in the Scroll View’s Content.
- Loop through the sorted list, and for each entry:
- Instantiate my score entry Prefab.
- Set the text of the Prefab to show the player’s name and score.
- Set the parent of the Prefab to the Scroll View’s Content.
Testing it Out
I added some dummy scores in the Start function, just to see if it was working. And… it did! I saw my list of scores, nicely sorted, in the Scroll View.
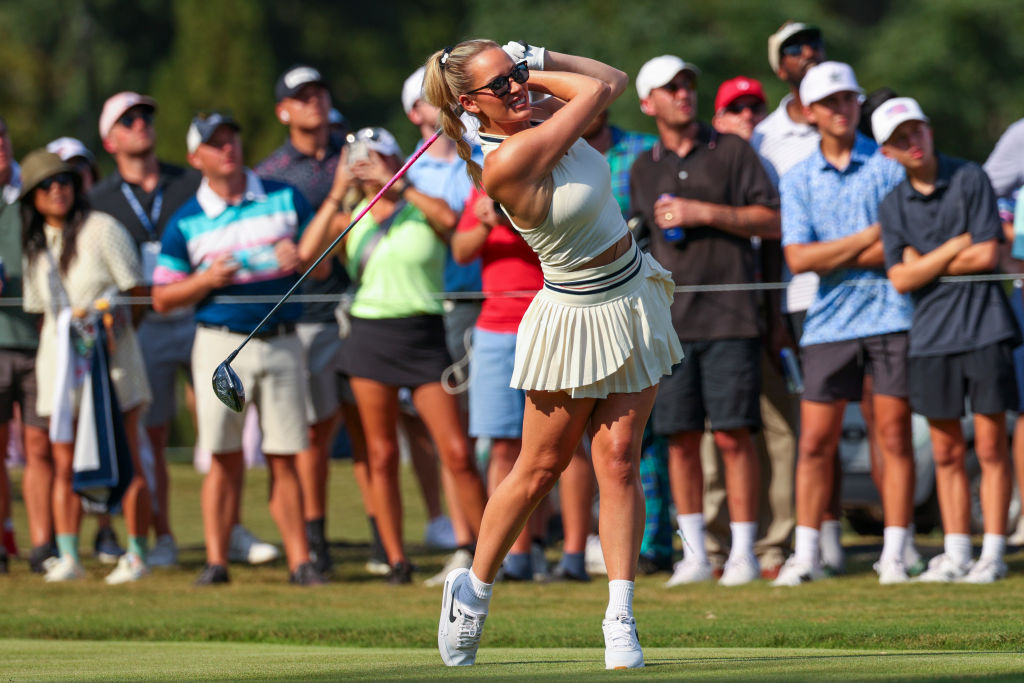
I added input, I used a simple button, when I click that button, simulate to add one score and refresh the Leaderboard.
Tweaking and Polishing
Of course, there’s always more you can do. I spent some time tweaking the layout, changing the colors, and making sure it looked good. I also added some error handling, just in case something went wrong. For example, making sure the player’s name wasn’t empty.
And that’s pretty much it! It’s a super simple leaderboard, but it does the job. It’s not perfect, but it’s a good starting point. And hey, it was way easier than I thought it would be!